菜鸟翻译(YIChangKong)
Gang of Four Design Patterns(设计模式四门派)
OverView(概述)
Over 20 years ago the iconic computer science book “Design Patterns: Elements of Reusable Object-Oriented Software” was first published.
The four authors of the book: Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, have since been dubbed “The Gang of Four”. In technology circles, you’ll often see this nicknamed shorted to GoF. Even though the GoF Design Patterns book was published over 20 years ago, it still continues to be an Amazon best seller.
20多年前,标志性的计算机科学著作“设计模式:可重用的面向对象软件的元素”首次出版。关于这个本书的四位作者: Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides,此后被成为“四人帮” ,在技术界,您经常会看到这个昵称,简称GoF。即使GoF Design Patterns一书出版了20多年,它仍然继续是亚马逊的畅销书。
The GoF wrote the book in a C++ context but it still remains very relevant to Java programming. C++ and Java are both object-oriented languages. The GoF authors, through their experience in coding large scale enterprise systems using C++, saw common patterns emerge. These design patterns are not unique to C++. The design patterns can be applied in any object oriented language.
GoF用c++编写了这本书,但它仍然与Java编程非常相关。c++和Java都是面向对象的语言。GoF的作者通过使用c++编写大型企业系统的经验,看到了常用模式的出现。这些设计模式并不是c++独有的。设计模式可以应用于任何面向对象语言。(扩展:C++ is a combination of both procedural and object-oriented programming languages.)
As a Java developer using the Spring Framework to develop enterprise class applications, you will encounter the GoF Design Patterns on a daily basis.
作为一名使用Spring框架开发企业级应用程序的Java开发人员,您每天(daily basis)都会遇到GoF设计模式。
The GoF Design Patterns are broken into three categories: Creational Patterns for the creation of objects; Structural Patterns to provide relationship between objects; and finally, Behavioral Patterns to help define how objects interact.
GoF设计模式可分为三类:用于创建对象的创建模式;提供对象之间关系的结构模式;最后,行为模式帮助定义对象如何交互。
Creational Design Patterns(创建型模式)
Abstract Factory. Allows the creation of objects without specifying their concrete type.
抽象工厂模式:允许在不指定对象具体类型(对象类型)的情况下创建对象
Builder. Uses to create complex objects.
建造者模式(Builder):用于创建复杂对象。
Factory Method. Creates objects without specifying the exact class to create.
工厂方法模式:创建对象,而不指定要创建的确切类。
Prototype.(原型) Creates a new object from an existing object.
原型模式(Prototype):从现有对象创建新对象。
Singleton. Ensures only one instance of an object is created.
单例模式(Singleton):确保只创建对象的一个实例。
Structural Design Patterns(结构型模式)
Adapter. Allows for two incompatible classes to work together by wrapping an interface around one of the existing classes.
适配器模式(Adapter):允许两个不兼容的类通过在一个现有类周围包装一个接口一起工作。
Bridge. Decouples an abstraction so two classes can vary independently.
桥接模式(Bridge):解耦一个抽象,这样两个类可以独立变化。(抽象分离??)
Composite. Takes a group of objects into a single object.
组合模式(Composite):将一组对象转换为单个对象
Decorator. Allows for an object’s behavior to be extended dynamically at run time.
装饰模式(Decorator)**装饰模式(Decorator)**:允许对象在运行时动态扩展对象的行为。
Facade. Provides a simple interface to a more complex underlying object.
外观模式(Facade):为更复杂的底层对象提供一个简单的接口。
Flyweight. Reduces the cost of complex object models.
(轻量化)享元模式(Flyweight):降低复杂对象模型的成本(任务?)。
Proxy. Provides a placeholder interface to an underlying object to control access, reduce cost, or reduce complexity.
代理模式(Proxy):为基础对象提供占位符接口,以控制访问、降低成本或降低复杂性。
Behavior Design Patterns(行为型模式)
Chain of Responsibility. Delegates commands to a chain of processing objects.
职责链模式(Chain of Responsibility): 将命令委托给处理对象链。
Command. Creates objects which encapsulate actions and parameters.
命令模式(Command):创建一个封装动作和参数的对象
Interpreter. Implements a specialized language.
解释器模式(Interpreter):实现一种特定的语言
Iterator. Accesses the elements of an object sequentially without exposing its underlying representation.
迭代器模式(Iterator):按顺序访问对象的元素,而不公开其底层表示。(sequencetially 按顺序)
Mediator. Allows loose coupling between classes by being the only class that has detailed knowledge of their methods.
中介者模式(Mediator):通过成为唯一详细了解其方法的类,实现允许类之间的松耦合。
Memento. Provides the ability to restore an object to its previous state.
备忘录模式(Memento):提供将对象恢复到以前状态的能力。
Observer. Is a publish/subscribe pattern which allows a number of observer objects to see an event.
观察者模式(Observer):是一个发布/订阅模式,它允许多个观察者对象查看事件。
State. Allows an object to alter its behavior when its internal state changes.
状态模式(State):允许对象在其内部状态改变时改变其行为。
Strategy. Allows one of a family of algorithms to be selected on-the-fly at run-time.
策略模式(Strategy):允许一个算法族在运行时被选中。
Template Method. Defines the skeleton of an algorithm as an abstract class, allowing its sub-classes to provide concrete behavior.
模板方法模式(Template Method):将算法的骨架定义为抽象类,从而允许其子类提供具体的行为
Visitor. Separates an algorithm from an object structure by moving the hierarchy of methods into one object.
访问者模式(Visitor):通过将方法的层次结构移动到一个对象中,将一种算法与一种对象结构分离。
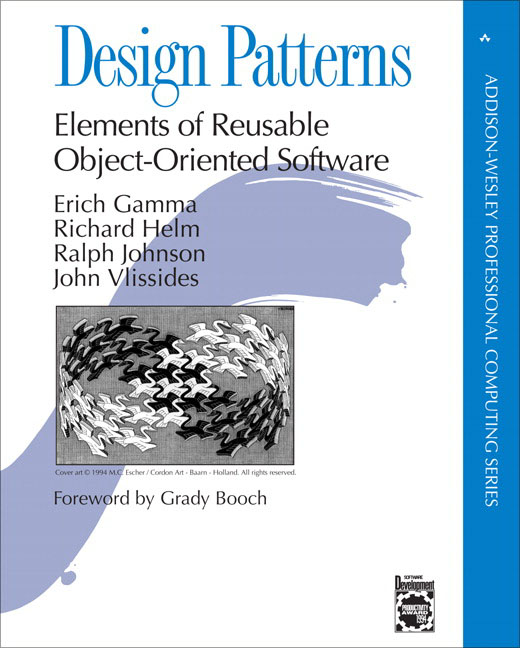