>[success] # defineProps
`defineProps` 作为编译器宏几个用法特点
* 不需要被导入即可使用,它会在编译`<script setup>`语法块时一同编译掉
* 只能在`<script setup>`中使用
* 接收与`props`选项相同的值
* 必须在`<script setup>`的顶层使用,不可以在`<script setup>`的局部变量中引用。
* `defineProps()`宏中的参数不可以访问`<script setup>`中定义的其他变量,因为在编译时整个表达式都会被移到外部的函数中。
1. 不需要被导入即可使用,它会在编译`<script setup>`语法块时一同编译掉
~~~html
<template> {{ props.name }} </template>
<!--在页面可以直接使用。但注意仅限页面-->
<template> {{ name }} </template>
<script setup>
// 无需导入直接使用
const props = defineProps({
name: String,
});
</script>
<style></style>
~~~
2. 接收与`props`选项相同的值
~~~ html
<template> {{ props.name }} </template>
<script setup>
import props from '../Props/props';
// const props = defineProps(['name']);
/**
* 支持配置字段 type 类型,required 是否必填,default默认值,validator 校验
* interface PropOptions<T> {
* type?: PropType<T>
* required?: boolean
* default?: T | ((rawProps: object) => T)
* validator?: (value: unknown) => boolean
* }
*
*/
// const props = defineProps({ name: String }); // 设置类型
const props = defineProps({
name: {
type: String,
require: true,
default: '1',
validator: (value) => {
return value.length > 1;
},
},
});
</script>
<style></style>
~~~
3. 必须在`<script setup>`的顶层使用,不可以在`<script setup>`的局部变量中引用。
~~~html
<template> {{ a.name }} </template>
<script setup>
// 报错不能在局部变量中使用:ReferenceError: defineProps is not defined
function aFun() {
const props = defineProps({
name: {
type: String,
require: true,
default: '1',
validator: (value) => {
return value.length > 1;
},
},
});
return props;
}
const a = aFun();
</script>
<style></style>
~~~
4. `defineProps()` 宏中的参数不可以访问 `<script setup>` 中定义的其他变量,因为在编译时整个表达式都会被移到外部的函数中。
~~~html
<template> {{ props.name }} </template>
<script setup>
const len = 1;
const props = defineProps({
name: {
type: String,
require: true,
default: '1',
validator: (value) => {
// 报错 `defineProps` are referencing locally declared variables
return value.length > len;
},
},
});
</script>
<style></style>
~~~
5. 只能在`<script setup>`中使用
* 在其他文件声明了一个`defineProps`
~~~
import { defineProps } from 'vue';
export default defineProps({
name: {
type: String,
require: true,
default: '1',
validator: (value) => {
return value.length > 1;
},
},
});
~~~
* 使用报错
~~~html
<template>
<div>测试</div>
<children :name="1" />
</template>
<script setup>
// 警告defineProps() is a compiler-hint helper that is only usable inside <script setup> of a single file component. Its arguments should be compiled away and passing it at runtime has no effect.
import children from './components/children.vue';
</script>
<style></style>
~~~
>[danger] ##### 其他配置项
1. 所有 prop 默认都是可选的,除非声明了`required: true`。
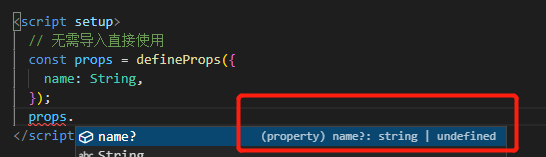
2. 未传递的 prop 会有一个缺省值`undefined`
3. 如果声明了`default`值,那么在 prop 的值被解析为`undefined`时,无论 prop 是未被传递还是显式指明的`undefined`,都会改为`default`值。
4. 声明多类型
~~~
defineProps({
disabled: [Boolean, Number]
})
~~~
5. 可以检测的类型,`String` `Number` `Boolean` `Array` `Object` `Date` `Function` `Symbol` `自定义类`
* 自定义类举例子
~~~
class Person {
constructor(firstName, lastName) {
this.firstName = firstName
this.lastName = lastName
}
}
defineProps({
author: Person
})
~~~
>[danger] ##### 使用技巧
1. **prop 被用于传入初始值;而子组件想在之后将其作为一个局部数据属性。** 在这种情况下,最好是新定义一个局部数据属性,从 prop 上获取初始值即可:
~~~
const props = defineProps(['initialCounter'])
// 计数器只是将 props.initialCounter 作为初始值
// 像下面这样做就使 prop 和后续更新无关了
const counter = ref(props.initialCounter)
~~~
2. **prop 以原始的形式传入,但还需作转换。** 在这种情况中,最好是基于该 prop 值定义一个计算属性:
~~~
const props = defineProps(['size'])
// 该 prop 变更时计算属性也会自动更新
const normalizedSize = computed(() => props.size.trim().toLowerCase())
~~~
>[info] ## 官网
[Props](https://cn.vuejs.org/guide/components/props.html)
- 官网给的工具
- 声明vue2 和 vue3
- 指令速览
- Mustache -- 语法
- v-once -- 只渲染一次
- v-text -- 插入文本
- v-html -- 渲染html
- v-pre -- 显示原始的Mustache标签
- v-cloak -- 遮盖
- v-memo(新)-- 缓存指定值
- v-if/v-show -- 条件渲染
- v-for -- 循环
- v-bind -- 知识
- v-bind -- 修饰符
- v-on -- 点击事件
- v-model -- 双向绑定
- 其他基础知识速览
- 快速使用
- 常识知识点
- key -- 作用 (后续要更新)
- computed -- 计算属性
- watch -- 侦听
- 防抖和节流
- vue3 -- 生命周期
- vue-cli 和 vite 项目搭建方法
- vite -- 导入动态图片
- 组件
- 单文件组件 -- SFC
- 组件通信 -- porp
- 组件通信 -- $emit
- 组件通信 -- Provide / Inject
- 组件通信 -- 全局事件总线mitt库
- 插槽 -- slot
- 整体使用案例
- 动态组件 -- is
- keep-alive
- 分包 -- 异步组价
- mixin -- 混入
- v-model-- 组件
- 使用计算属性
- v-model -- 自定义修饰符
- Suspense -- 实验属性
- Teleport -- 指定挂载
- 组件实例 -- $ 属性
- Option API VS Composition API
- Setup -- 组合API 入口
- api -- reactive
- api -- ref
- 使用ref 和 reactive 场景
- api -- toRefs 和 toRef
- api -- readonly
- 判断性 -- API
- 功能性 -- API
- api -- computed
- api -- $ref 使用
- api -- 生命周期
- Provide 和 Inject
- watch
- watchEffect
- watch vs. watchEffect
- 简单使用composition Api
- 响应性语法糖
- css -- 功能
- 修改css -- :deep() 和 var
- Vue3.2 -- 语法
- ts -- vscode 配置
- attrs/emit/props/expose/slots -- 使用
- props -- defineProps
- props -- defineProps Ts
- emit -- defineEmits
- emit -- defineEmits Ts
- $ref -- defineExpose
- slots/attrs -- useSlots() 和 useAttrs()
- 自定义指令
- Vue -- 插件
- Vue2.x 和 Vue3.x 不同点
- $children -- 移除
- v-for 和 ref
- attribute 强制行为
- 按键修饰符
- v-if 和 v-for 优先级
- 组件使用 v-model -- 非兼容
- 组件
- h -- 函数
- jsx -- 编写
- Vue -- Router
- 了解路由和vue搭配
- vueRouter -- 简单实现
- 安装即使用
- 路由懒加载
- router-view
- router-link
- 路由匹配规则
- 404 页面配置
- 路由嵌套
- 路由组件传参
- 路由重定向和别名
- 路由跳转方法
- 命名路由
- 命名视图
- Composition API
- 路由守卫
- 路由元信息
- 路由其他方法 -- 添加/删除/获取
- 服务器配置映射
- 其他
- Vuex -- 状态管理
- Option Api -- VUEX
- composition API -- VUEX
- module -- VUEX
- 刷新后vuex 数据同步
- 小技巧
- Pinia -- 状态管理
- 开始使用
- pinia -- state
- pinia -- getter
- pinia -- action
- pinia -- 插件 ??
- Vue 源码解读
- 开发感悟
- 练手项目