SQLite3 可使用 sqlite3 模块与 Python 进行集成。sqlite3 模块是由 Gerhard Haring 编写的。它提供了一个与 PEP 249 描述的 DB-API 2.0 规范兼容的 SQL 接口。您不需要单独安装该模块,因为 Python 2.5.x 以上版本默认自带了该模块,所以使用时直接导入即可。
sqlite的基础教程可以参考SQLite - Python官方文档下面举一个简单的例子:1、插入数据rollback()用于事务回滚;出现错误时可以使用回滚;
#coding:utf-8
import sqlite3
#使用connect()方法创建或打开数据库,指定数据库的路径,如果存在直接打开,不存在则创建一个新的数据库;
con = sqlite3.connect('E:\\sqlite\\test.db')#con = sqlite3.connect(':memory:') 在内存中创建数据库
#通过cursor方法创建一个游标对象
cur = con.cursor()
#创建表person
cur.execute(' CREATE TABLE person (id integer primary key,name varchar(20),age integer)')
#插入数据
data="0,'sara',20"
cur.execute(' INSERT INTO person VALUES (%s)'%data) #直接构造插入的SQL的语句,但是这种不安全,容易导致SQL注入,可以使用占位符“?”规避
cur.execute(' INSERT OR IGNORE INTO person VALUES (?,?,?)',(1,'迈克',24)) #使用占位符“?”更安全
#使用executemany执行多条SQL语句,使用executeman()比循环使用execute()使用高效
cur.executemany(' INSERT INTO person VALUES (?,?,?)',[(2,'开心麻花',30),(3,'jack',50)])
#插入数据不会立即生效,需要commit()提交;
con.commit()
#关闭数据库连接
con.close()
执行结果:
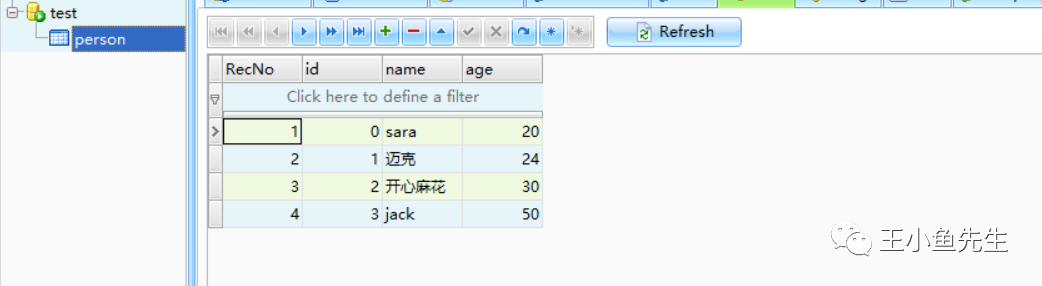
2、查询数据fetchone()从结果中取一条记录,并将游标指向下一条记录;fetchmany()从结果中取多条记录;fetchall()从结果中取出全部记录;scroll()用于游标滚动;
1)查询全部数据
#coding:utf-8
import sqlite3
#使用connect()方法创建或打开数据库,指定数据库的路径,如果存在直接打开,不存在则创建一个新的数据库;
con = sqlite3.connect('E:\\sqlite\\test.db')#con = sqlite3.connect(':memory:') 在内存中创建数据库
#通过cursor方法创建一个游标对象
cur = con.cursor()
#创建表person
cur.execute(' CREATE TABLE person (id integer primary key,name varchar(20),age integer)')
#插入数据
data="0,'sara',20"
cur.execute(' INSERT INTO person VALUES (%s)'%data) #直接构造插入的SQL的语句,但是这种不安全,容易导致SQL注入,可以使用占位符“?”规避
cur.execute(' INSERT OR IGNORE INTO person VALUES (?,?,?)',(1,'迈克',24)) #使用占位符“?”更安全
#使用executemany执行多条SQL语句,使用executeman()比循环使用execute()使用高效
cur.executemany(' INSERT INTO person VALUES (?,?,?)',[(2,'开心麻花',30),(3,'jack',50)])
# 查询表person的所有数据
cur.execute('SELECT * FROM person')
#fetchall()获取所有数据,返回一个二维的列表
res = cur.fetchall()
print(res)
for line in res:
print(line)
#插入数据不会立即生效,需要commit()提交;
con.commit()
#关闭数据库连接
con.close()
执行结果:
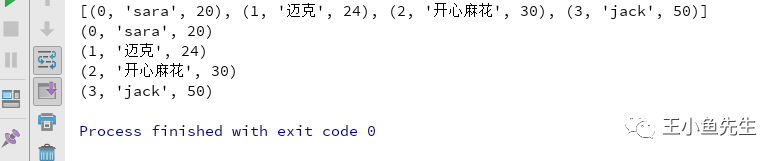
2)查询单个数据
#coding:utf-8
import sqlite3
#使用connect()方法创建或打开数据库,指定数据库的路径,如果存在直接打开,不存在则创建一个新的数据库;
con = sqlite3.connect('E:\\sqlite\\test.db')#con = sqlite3.connect(':memory:') 在内存中创建数据库
#通过cursor方法创建一个游标对象
cur = con.cursor()
#创建表person
cur.execute(' CREATE TABLE person (id integer primary key,name varchar(20),age integer)')
#插入数据
data="0,'sara',20"
cur.execute(' INSERT INTO person VALUES (%s)'%data) #直接构造插入的SQL的语句,但是这种不安全,容易导致SQL注入,可以使用占位符“?”规避
cur.execute(' INSERT OR IGNORE INTO person VALUES (?,?,?)',(1,'迈克',24)) #使用占位符“?”更安全
#使用executemany执行多条SQL语句,使用executeman()比循环使用execute()使用高效
cur.executemany(' INSERT INTO person VALUES (?,?,?)',[(2,'开心麻花',30),(3,'jack',50)])
#fetchone()获取其中的一个结果,返回一个元组
cur.execute('SELECT * FROM person')
res = cur.fetchone()
print(res)
#插入数据不会立即生效,需要commit()提交;
con.commit()
#关闭数据库连接
con.close()
执行结果:

3、修改和删除数据
#coding:utf-8
import sqlite3
#使用connect()方法创建或打开数据库,指定数据库的路径,如果存在直接打开,不存在则创建一个新的数据库;
con = sqlite3.connect('E:\\sqlite\\test.db')#con = sqlite3.connect(':memory:') 在内存中创建数据库
#通过cursor方法创建一个游标对象
cur = con.cursor()
#创建表person
cur.execute(' CREATE TABLE person (id integer primary key,name varchar(20),age integer)')
#插入数据
data="0,'sara',20"
cur.execute(' INSERT INTO person VALUES (%s)'%data) #直接构造插入的SQL的语句,但是这种不安全,容易导致SQL注入,可以使用占位符“?”规避
cur.execute(' INSERT OR IGNORE INTO person VALUES (?,?,?)',(1,'迈克',24)) #使用占位符“?”更安全
#使用executemany执行多条SQL语句,使用executeman()比循环使用execute()使用高效
cur.executemany(' INSERT INTO person VALUES (?,?,?)',[(2,'开心麻花',30),(3,'jack',50)])
#修改数据
cur.execute('UPDATE person SET name=? WHERE id=?',('小明',1))
#删除数据
cur.execute('DELETE FROM person WHERE id=?',(0,))
#插入数据不会立即生效,需要commit()提交;
con.commit()
#关闭数据库连接
con.close()
执行结果:
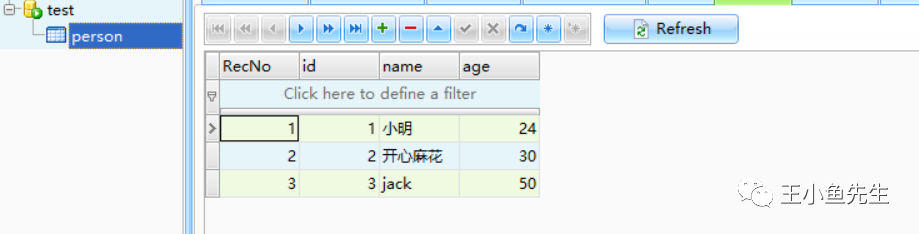
特别注意:1、执行完数据库操作后必须要关闭数据库;2、插入或修改中文数据时,如果出现乱码,可尝试在中文字符串前加“u”
下面是用之前天气的例子存到sqlite
import requests
from lxml import etree
import json
import sqlite3
#使用connect()方法创建或打开数据库,指定数据库的路径,如果存在直接打开,不存在则创建一个新的数据库;
con = sqlite3.connect('E:\\sqlite\\tianqi.db')#con = sqlite3.connect(':memory:') 在内存中创建数据库
#通过cursor方法创建一个游标对象
cur = con.cursor()
#创建表person
cur.execute(' CREATE TABLE shenzhen (date,date2,history_rain,history_max,history_min,tmax,tmin,time,w1,wd1,alins,als)')
class Weather():
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36',
'Referer': 'http://www.weather.com.cn/weather40d/101280601.shtml'
}
# 新建列表,用来存储获取的数据
all_info = []
def __init__(self):
pass
# 获取一年的天气数据
def get_data(self):
global year
month = ['01', '02', '03', '04', '05', '06', '07', '08', '09', '10', '11', '12']
for i in month:
url = 'http://d1.weather.com.cn/calendar_new/' + str(year) + '/101280601_' + str(year) + str(
i) + '.html?_=1496558858156'
html = requests.get(url, headers=self.headers).content
global datas
datas = json.loads(html[11:])
self.get_info()
# 获取天气的具体数据
def get_info(self):
for data in datas:
if data['date'][:4] == year:
date = data['date']
date2 = data['nlyf'] + data['nl']
history_rain = data['hgl']
history_max = data['hmax']
history_min = data['hmin']
tmax = data['max']
tmin = data['min']
time = data['time']
w1 = data['w1']
wd1 = data['wd1']
alins = data['alins']
als = data['als']
#先把去重的数据存储到列表all_info
info = (date,date2,history_rain,history_max,history_min,tmax,tmin,time,w1,wd1,alins,als )
if info not in self.all_info:
self.all_info.append(info)
#把数据存到sqlite
def store_sqlite(self):
self.get_data()
for one_info in self.all_info:
cur.execute(' INSERT OR IGNORE INTO shenzhen VALUES (?,?,?,?,?,?,?,?,?,?,?,?)',one_info)
con.commit()
if __name__ == '__main__':
year = '2017'
tianqi = Weather()
tianqi.store_sqlite()
# 关闭数据库连接
con.close()
运行结果:
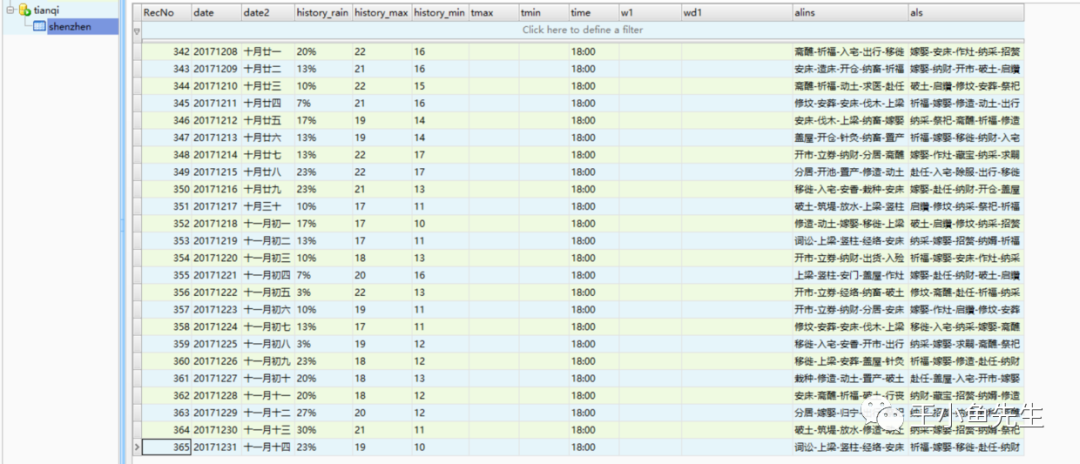
sqlite的可视化工具:SQLite Expert Professional
文章转载自王小鱼先生,如果涉嫌侵权,请发送邮件至:contact@modb.pro进行举报,并提供相关证据,一经查实,墨天轮将立刻删除相关内容。